DeFi TWAP: Uniswap and Chainlink
Mar 17, 2024
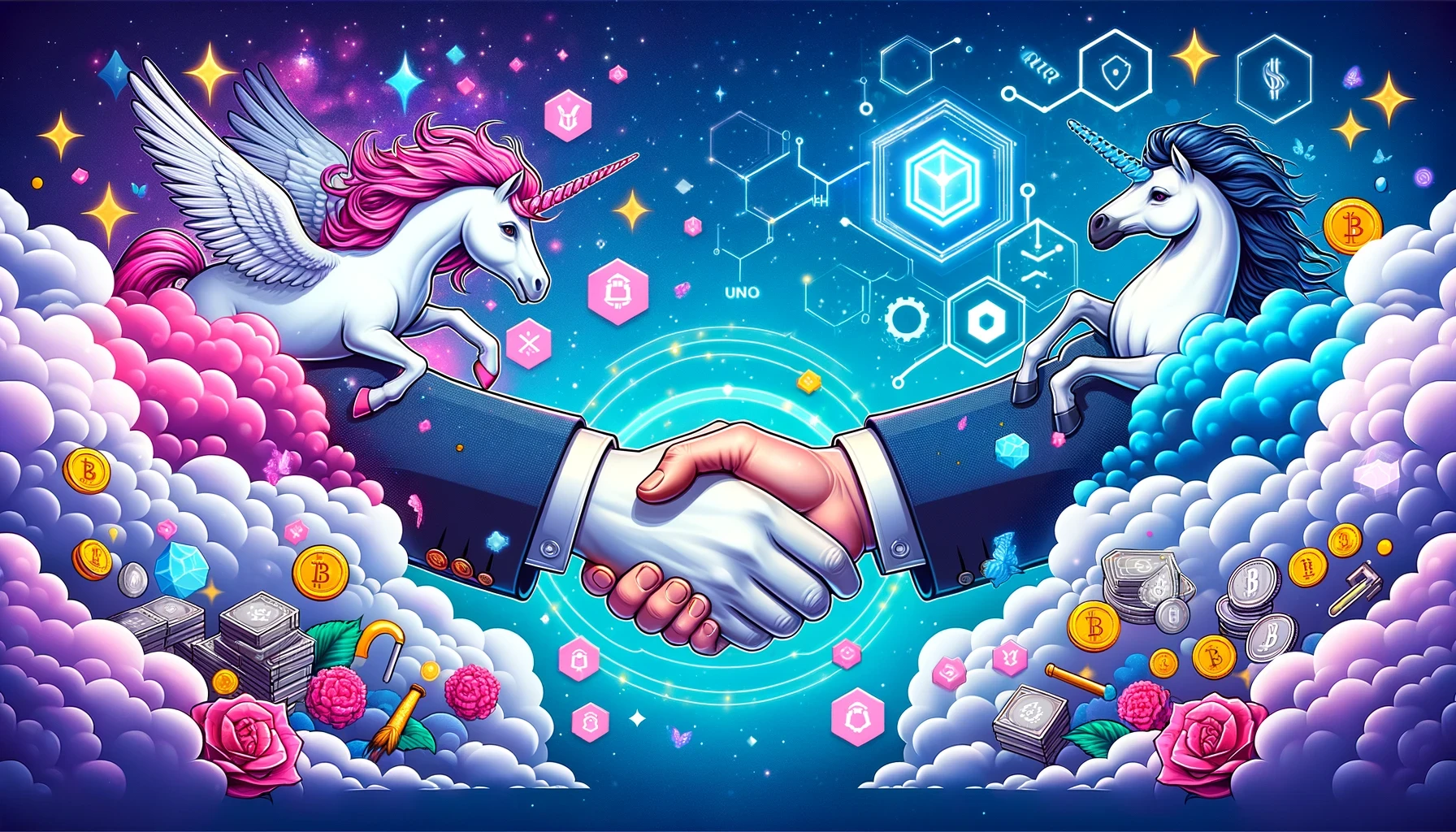
Background
Uniswap is the premier decentralized exchange with innovations such as concentrated liquidity and aggregated order routing. While it is possible to use the Uniswap User Interface to complete your trades, you might benefit from deploying your own smart contract which will perform trades on your behalf. Using Chainlink Automations, you can add logic to an upkeep function which is triggered by a trust-minimized, decentralized network on a predictable basis. For most people doing simple trades, the user interface is sufficient. But for complicated mandates or larger funds, some custom trade configuration could be beneficial.
TWAP or time-weighted average price is trade strategy which spreads out a single order in regular intervals over a period of time. Institutional trading products offer the execution of TWAP which achieves a near average execution price in the specified time range. This reduces execution risks like partial fill for limit orders and price or market manipulation. Institutional buyers are more likely to execute a trade over the course of a few hours or even a full day to capture what they believe is a fair price within the allotted time interval.
Present Value
The newly launched "Duncun" upgrade to the Ethereum network will reduce the cost of performing transactions on Layer-2 networks. This reduction in fees taken by the blockchain for performing operations will enable new kinds of frequency-based financial operations, such as diversified trade types.
In fact, many operations on Layer-2 networks will see costs reduced to nearly-zero; adding all but the highest-frequency trading techniques to the toolkit of decentralized funds. See an estimate from CoinBureau on X: https://twitter.com/coinbureau/status/1768660393684038108
Using the learnings of institutional traders in traditional finance, you can benefit from TWAP in decentralized trading by using verifiable, off-chain execution network like those offered by Chainlink Automations. This guide will show you how to implement this institutional trading strategy for your crypto fund and sharpen your edge.
Be cautious when creating smart contracts. You could lose any funds deposited to a security or requirement bug, so be cautious. This guide is for experienced DeFi traders and Solidity developers alike who want to execute sophisticated trading strategies using only decentralized infrastructure.
Technical Walkthrough
Let's start with a simple trade smart contract we'll call TWAP. This contract will be responsible for accepting deposit of the first token in our swap pair, performing the incremental fixed size trades, and ultimately allow withdrawal of the second token in our trading pair.
Let's first implement the depositToken and withdrawalToken functions
Then we will make a simple swap using the Uniswap ISwapRouter interface. In this implementation we use the ExactInputSingleParams function on the router, which allows us to specify an exact input value of one token for a variable output of another token, including a lower-bound amountOutMinimum and an explicit limit-price. A deadline setting of block.timestamp indicates that the swap should be done with atomicity.
It's helpful to understand the Ethereum network (and by extension the Layer-2 scaling solutions which settle ultimately on the network) as a stateful clock. The network does not have the capability to execute transactions on your behalf. There are solutions such as the Ethereum Alarm Clock and now Chainlink Automations to perform actions on our behalf.
We will define a TimedSwapUpkeep contract which solve our caller-source issue using decentralized infrastructure provided by Chainlink. To properly interact with Chainlink automation we must implement two functions, a checkUpkeep and performUpkeep. You can find out more about the necessary interface here: https://docs.chain.link/chainlink-automation/guides/compatible-contracts
The deployment of the contract to the Layer-2 scaling solution of your choice is simple, see this example for Arbitrum:
Once you have deployed your contracts to an EVM-compatible network, you'll navigate to the Chainlink Automation registration page: https://functions.chain.link/mainnet and register a new "time-based upkeep".
This upkeep will contact your checkUpkeep function on the agreed upon cron schedule string. Following the patterns of institutional traders, we may opt for a 10-minute execution pattern which is expressed in cron as
Although you may choose any pattern which meets your trading needs.
Next, fund your upkeep with a few LINK tokens which will pay a for use of the decentralized keeper network and gas fees of executing the functions on your smart contracts. Recall that fees will see a large reduction with the newly deployed Dencun upgrade, so beware of the savings you'll encounter and fund upkeeps accordingly.
At the end of the deployment and registration, you'll have a fully autonomous TWAP trade executor which will begin performing swaps at the next upkeep the TWAP contract is funded with the first token in your trading pair. See the repo for full code examples with mock tokens, which work better on testnet https://github.com/irasigman/defi-twap
Afterwards
If you liked what you read here, follow me on X at @0x1ra or @0xIra on Farcaster.